CollapsingToolbarLayout을 이용하여 확장/축소되는 툴바에 대해서 알아보겠습니다.
아래 애니메이션과 같이, 스크롤을 위로하면 일반 툴바로 변하고, 아래로 움직이면 확장된 툴바가 보이는 UI 입니다.
개발은 기존에 리스트 샘플로 개발했던 activiy에 추가 개발로 진행하겠습니다.
Paging 3.0 + MVVM + Flow를 이용하여 리스트 구현하기
앱을 개발하면 빠지지않고 사용하는 페이징 리스트를 구현해 보도록 하겠습니다. 자동으로 페이징을 해주는 Paging 3.0 라이브러리를 이용하여 MVVM 모델과 Flow를 사용하여 연동할 예정입니다. 지
heeeju4lov.tistory.com
1. activity_lorempicsum_list.xml 파일에 확장/축소 툴바로 사용될 widget 코드를 추가합니다.
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:fitsSystemWindows="true">
<com.google.android.material.appbar.CollapsingToolbarLayout
android:layout_width="match_parent"
android:layout_height="240dp"
android:fitsSystemWindows="true"
app:contentScrim="@color/purple_700"
app:expandedTitleGravity="center"
app:layout_scrollFlags="scroll|exitUntilCollapsed|snap"
app:scrimVisibleHeightTrigger="156dp"
app:statusBarScrim="@android:color/transparent"
app:expandedTitleTextAppearance="@style/CollapsingToolbar.Expanded.Title"
app:collapsedTitleTextAppearance="@style/CollapsingToolbar.Collapsed.Title">
<androidx.appcompat.widget.AppCompatImageView
android:id="@+id/iv_toolbar_background"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
app:url='@{"https://picsum.photos/200/300"}'
android:fitsSystemWindows="true"/>
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize">
</androidx.appcompat.widget.Toolbar>
</com.google.android.material.appbar.CollapsingToolbarLayout>
</com.google.android.material.appbar.AppBarLayout>
...
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</layout>
- CoordinatorLayout : 최상의 layout으로 스크롤이 있는 widget(RecyclerView, ScrollView 등등) 과 연동을 하여 동작합니다.
- AppBarLayout : 확장/축소 툴바 layout으로 listener를 통해서 확장/축소에 대한 event를 받을 수 있습니다.
- CollapsingToolbarLayout : 실제 툴바 widget으로 확장되었을때 툴바의 UI를 정의합니다.
- Toolbar : 축소되었을때의 기본 툴바 widget입니다.
간단하게 사용하는 widget에 대해서 설명 하였습니다.
이제 widget의 속성에 대해서 알아보겠습니다.
- android:fitsSystemWindowns="true" : 여러 widget에 공통적으로 들어가는 속성으로, 전체영역(status bar를 포함)을 사용하는 속성입니다.
이 속성을 사용하려먼 Activity에 테마를 설정해 주어야 합니다.
먼저, themes.xml에 파일에 statusBar 의 배경색을 투명으로 정의하는 style를 추가합니다.
style에 parent 는 필히 NoActionBar를 사용해야 합니다.
<!-- theme of CollapsingToolbar toolbar -->
<style name="Theme.SampleApp.CollapsingToolbar" parent="Theme.AppCompat.DayNight.NoActionBar">
<item name="android:statusBarColor">@android:color/transparent</item>
</style>
그리고, 추가한 style을 AndroidManifest.xml 파일에 Activity에 theme로 추가해 줍니다.
<activity
android:name=".lorem_picsum.ui.LoremPicsumListActivity"
android:theme="@style/Theme.SampleApp.CollapsingToolbar"/>
- app:contentsScrim="@color/purple_700" : 툴바가 축소되었을때의 배경색을 정의합니다.(저는 퍼플로 했어요)
- app:expandedTitleGravity="center" : 툴바가 확장되었을때, 타이틀의 정렬 위치입니다.
- app:layout_scrollFlags="scroll|exitUntilCollapsed|snap" : 툴바의 확장/축소시의 동작에 대해 정의합니다. 정의된 설정은 스크롤 위로 움직이는 경우 기본 툴바를 표시하고, 스크롤을 내릴때는 스크롤이 모두 내려오고 툴바가 확장되도록 하였습니다.(더 많은 설정은 구글링을 해보시면 많이 나옵니다.)
- app:scrimVisibleHeightTrigger="156dp" : 확장된 툴바가 축소될때 어느 위치에 왔을때 축소 UI가 시작될지를 정의합니다.
- app:statusBarscrim="@android:color/transparent" : 툴바가 축소가 되었을때 statusBar의 배경색입니다. 저는 투명을 주었는데, 색을 넣는 경우엔, 툴바의 배경색과 statusBar의 배경색이 나타나는 애니메이션 타이밍이 달라서 약간 어색한 느낌이 들어서, 투명으로 하였습니다.
- app:expandedTitleTextAppearance="@style/CollapsingToolbar.Expanded.Title" : 확장된 툴바의 타이틀 텍스트의 속성을 정의합니다.
- app:collapsedTitleTextAppearance="@style/CollapsingToolbar.Collapsed.Title" : 축소된 툴바의 타이틀 텍스트의 속성을 정의합니다.
themes.xml 파일에 CollapsingToolbar.Expanded.Title 와 CollapsingToolbar.Collapsed.Title 정의
<style name="CollapsingToolbar.Expanded.Title" parent="@android:style/TextAppearance">
<item name="android:textColor">@android:color/white</item>
<item name="android:textSize">32sp</item>
</style>
<style name="CollapsingToolbar.Collapsed.Title" parent="@android:style/TextAppearance">
<item name="android:textColor">@android:color/white</item>
<item name="android:textSize">24sp</item>
</style>
CollapsingToolbarLayout 하위로 ImageVIew와 Toolbar widget을 보실 수 있으십니다.
- ImageView는 확장되었을때 배경이미지를 표시합니다.
- Toolbar는 축소되었을때 표시되는 툴바입니다.
예제에서는 간단하게 표현하였지만, 실제 프로젝트에서는 layout이나 버튼, 뷰 등 다양한 widget을 추가하여 개발하시면 됩니다.
이제 개발 코드에서 Activity의 ActionBar와 연동을 연결해주면 됩니다.
LoremPicsumListActivity.kt 의 initView()에 아래 코드를 추가합니다.
(저는 BaseActivity를 사용하므로 initView()에 추가하였지만, 일반적으로는 onCreate() 에 추가하시면 됩니다.)
setSupportActionBar(binding.toolbar)
supportActionBar?.setDisplayShowTitleEnabled(true)
title = "CollapsingToolbar"
위의 코드는 activity의 기본 actionBar 를 layout xml 에서 정의한 toolbar로 사용하고, actionBar의 타이틀을 그대로 사용하겠다는 코드입니다.
그리고, 툴바의 title을 추가해 주었습니다.
이렇게해서 모든 개발은 끝났습니다.
이제 실행하시면 맨위에 애니메이션처럼 확대/축소되는 툴바를 보실 수 있으십니다.
전체 개발 코드는 아래 Github 링크를 확인해주세요.
GitHub - rcbuilders/RemindSampleApp: https://heeeju4lov.tistory.com/ 블로그에서 Android + Kotlin 강좌에서 사용함.
https://heeeju4lov.tistory.com/ 블로그에서 Android + Kotlin 강좌에서 사용함. - GitHub - rcbuilders/RemindSampleApp: https://heeeju4lov.tistory.com/ 블로그에서 Android + Kotlin 강좌에서 사용함.
github.com
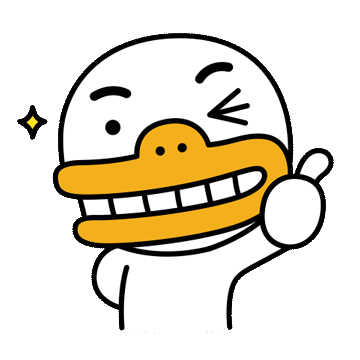
'Android + Kotlin' 카테고리의 다른 글
[Android + Kotlin] PagingDataAdapter에서 "좋아요"를 해보자. (payload update) (0) | 2022.08.29 |
---|---|
[Android + Kotlin] Shimmer 라이브러리를 이용하여 로딩 화면 만들기 (0) | 2022.08.10 |
[Android + Kotlin] Android Studio에서 Release로 빌드하여 단말에 실행하기 (0) | 2022.06.16 |
[android + Kotlin] Runtime에 View 속성 확인 라이브러리 (for 디자이너 협업) (0) | 2022.05.30 |
[Android + Kotlin] startActivityForResult 의 Deprecated 를 해결하자. (0) | 2022.05.10 |